For better understanding, I am providing a scenario based example
Scenario: Let's say you have a project and your project has different libraries on which your project depends and each library has its own prerequisites / dependent libraries. So what will be your approach to handle such kind of dependency. The simple approach will be to have a file with library names and their dependents in the form of a following tree, so that you can remember for future.
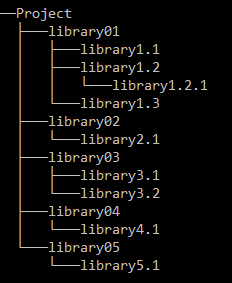
Now that you have installed your project dependencies and structure looks like the above one. What if you have to shift the project to another location or you want to have the same project to be made 2-3 years later. That's ok, you will just copy the above project with its dependencies and paste it there, isn't it simple enough. Yes, but there are some things that need to be taken care of:
- What if the libraries you used in the first project are deprecated now.
- What if the libraries have new versions with additional features.
- You have to check each library whether it has new version available.
The situations like the above one, needs to be handled. There comes the Composer tool that handles such kinds of situation and do more than that.
In short: Composer is a tool for dependency management in PHP. Composer stores the libraries in a directory usually named vendor in your project.
Let's do the practical work and usage of composer:
If you need to use composer in your project, the file you need is composer.json
. It is just a text file that lists package names and versions. What this file does is maintain your dependencies.
Let's look into the composer.json file.
{
"require" : {
"vendor/package" : "1.0.1"
}
}
You then run the composer program that reads this file and then it downloads and installs the packages.
Following commands serves the purpose of downloading and installing the packages:
> composer install
If the package you want is not listed in the composer.json file and you want that to be installed and also included in composer.json file then following command is useful for this:
> composer require "vendor/package : 1.0.1"